PHP SDK
This document provides information on how to use the Hackle PHP SDK.
Before You Read
- If this is your first time working with an SDK, please refer to the What is a SDK? document
- Please use Hackle PHP SDK 1.0.0 version or later PHP-SDK
- We support PHP 7.1.33 or later
- There are several keys mentioned in this document. In order to find out where to obtain these keys and the roles of each key during the SDK integration, please read Keys Used by SDK Functions before you read this document.
Sequence of SDK Integration and Configuration
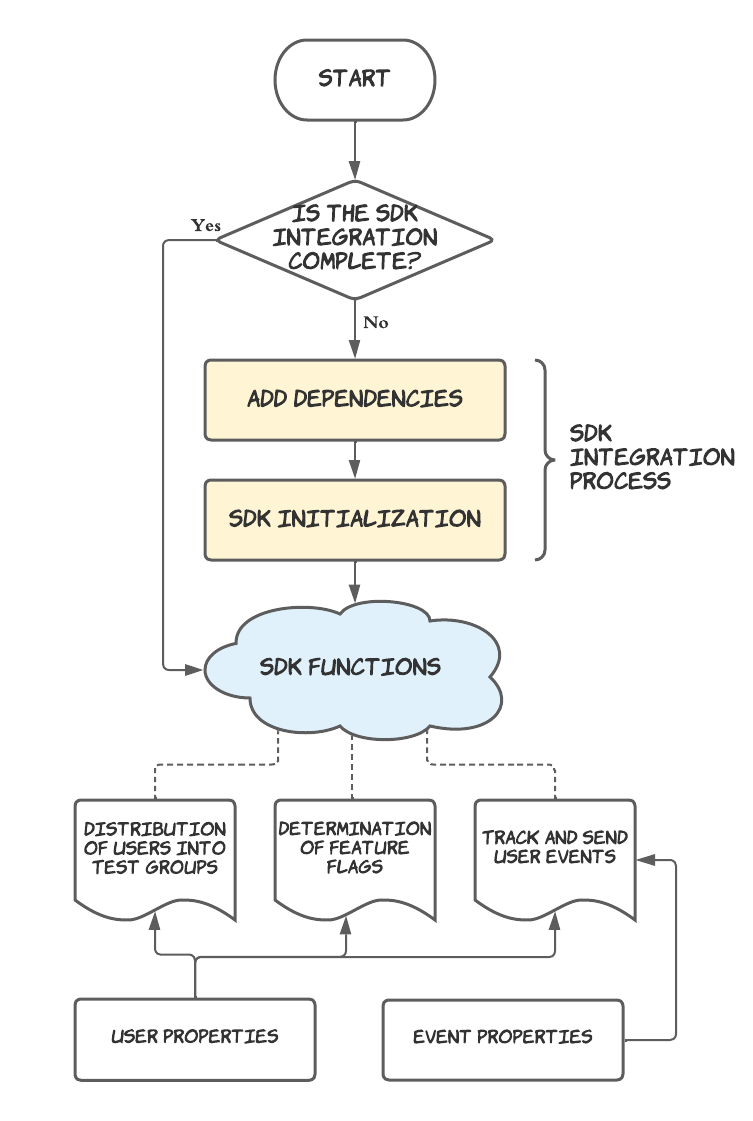
1. SDK Integration
You must integrate the SDK into your personal code in order to use Hackle's services.
You only have to do SDK Integration once. For example, if you are already tracking and sending events but would like to also use the feature flag function, you do not need to re-integrate a new SDK. Once integrated, you're all connected. Make sure you do not initialize the SDK multiple times, as this may lead to problems.
2. The Functions of SDK
Different types of SDK functions can be used together depending on the main purpose. This should also be set in advance before using the functions.
Some typical use cases include:
Case 1. Determining test groups for users in A/B Testing
This function distributes incoming user traffic into test groups A, B, and C and returns the information on the assigned groups of the users back to the server to keep a record of each userβs exposure after the distribution is complete. We also call this the "distribution of users into test groups" function.
After distribution, the SDK collects the user behavior accordingly to each test group. The user behavior is a pre-defined user event.
This step is necessary to calculate the metrics and findings of A/B testing.
Case 2. Retrieve feature flag values
If you apply a feature flag for a specific feature, you can enable or disable that feature with a single click on the Hackle Dashboard. The SDK needs to be able to get those values at the code level and consequently determine the on/off state of the feature flag for each user.
Case 3. Send Events
This is used to record the actions (events) of a specific user.
This function is used the most.
Because it records behavior, it can be useful for A/B testing or measuring responses to feature flags.
It can also be useful for logging, analyzing user funnels, and more.
Case 4. Data Segmentation Analysis
You can perform detailed data analysis using different user properties.
User properties can be given to user objects and sent together when distributing traffic into test groups / determining feature flags / sending user events.
Event properties can be assigned to an event, and can be sent together when tracking/ sending user events.
Case 5. Targeting
You can target users with specific properties in A/B tests and feature flags.
In the case of A/B testing, only users with specific properties can participate in A/B testing, and in the case of feature flags, you can apply the feature flags to users with specific properties.
Case 6. Remote Config
Remote config controls the behavior and settings of an application in real-time by replacing the values or properties controlled in application with the parameters defined in the hackle dashboard. The parameter can be set to a target value for all properties of the client/server side app (ex. button color, external link).
Updated over 1 year ago