Domain Proxy
This guide explains how to set up a proxy server using your own domain and use it with the Hackle SDK.
This is useful for reducing the impact of ad-blocking programs on tracking user behavior.
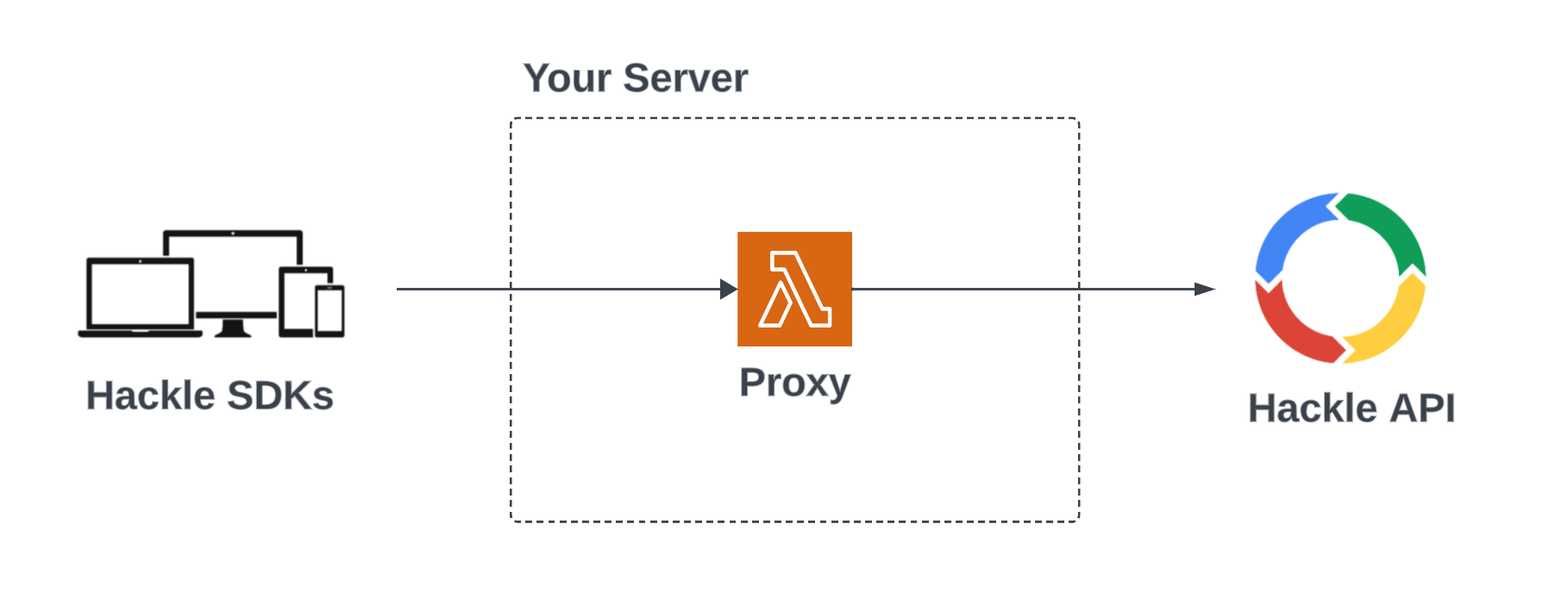
To set up and apply a proxy server, you need to configure the following two steps:
- Set up the proxy server
- Configure the SDK to point to the proxy server
1. Set up the proxy server
Setup via cloud
Most major cloud providers allow you to easily develop and deploy proxy services.
Here's an example of setting up a proxy service using AWS CloudFront.
-
Access the AWS CloudFront Console
-
Click "Create distribution"
-
Origin area
-
Set Origin domain to
event.hackle.io
-
Set Protocol to
HTTPS Only
-
-
Default cache behavior area
- Set Viewer protocol policy to
Redirect HTTP to HTTPS
- Set Allowed HTTP methods to
GET, HEAD, OPTIONS, PUT, POST, PATCH, DELETE
- Cache key and origin requests: Allow all Headers and Parameters to be passed to the Origin and allow CORS requests to the Origin.
- Set Viewer protocol policy to
[Image placeholder]
- In Function associations area
- Origin request: Add a Lambda@Edge Function to change the
Host
header toevent.hackle.io
.
Reference: Tutorial: Creating a simple Lambda@Edge function
- Origin request: Add a Lambda@Edge Function to change the
[Image placeholder]
Use the following code in the Lambda@Edge Function:
export const handler = (event, context, callback) => {
const request = event.Records[0].cf.request;
request.headers.host[0].value = 'event.hackle.io';
return callback(null, request);
};
- Click "Create distribution" at the bottom of the screen
Building a proxy server
You can also build a proxy server directly.
Here's an example of building a proxy server using NGINX. The following configuration redirects all calls to the proxy server to Hackle.
events {}
http {
server {
listen 80;
listen [::]:80;
location / {
proxy_set_header Host event.hackle.io;
proxy_set_header X-Real-IP $http_x_forwarded_for;
proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for;
proxy_set_header X-Forwarded-Host $server_name;
proxy_pass https://event.hackle.io/;
}
}
}
2. SDK Configuration
You need to configure the SDK to point to the proxy server. This can be set through the eventUrl
option when initializing the SDK.
const config = {
eventUrl: "https://<YOUR_PROXY_DOMAIN>"
};
createInstance(YOUR_SDK_KEY, config);
HackleConfig config = HackleConfig.builder()
.eventUri("https://<YOUR_PROXY_DOMAIN>")
.build();
HackleApp.initializeApp(getApplicationContext(), YOUR_SDK_KEY, config)
let config = HackleConfigBuilder()
.eventUrl(URL(string: "https://<YOUR_PROXY_DOMAIN>")!)
.build()
Hackle.initialize(sdkKey: YOUR_SDK_KEY, config: config)
HackleConfig config = HackleConfigBuilder()
.eventUrl("https://<YOUR_PROXY_DOMAIN>")
.build();
await HackleApp.initialize(YOUR_SDK_KEY, hackleConfig: config);
Updated 7 months ago